How to upload AVIF images in WordPress
AVIF format provides superior image quality at remarkably smaller file sizes, making it ideal for use on websites where optimizing file size is crucial.
However, WordPress does not support AVIF images natively, which means you cannot upload AVIF images to your WordPress site and expect them to work seamlessly.
In this article, we will address the issues related to uploading AVIF images to WordPress and provide solutions to overcome these challenges.
Uploading AVIF Images
By default, WordPress does not support uploading AVIF images. To rectify this, you need to include the AVIF format in WordPress’s list of supported mime types.
Here’s a code snippet that can be added to your theme to enable AVIF support:
add_filter( 'upload_mimes', 'filter_allowed_mimes_for_avif', 1000, 1 );
function filter_allowed_mimes_for_avif( $mime_types ) {
$mime_types['avif'] = 'image/avif';
return $mime_types;
}
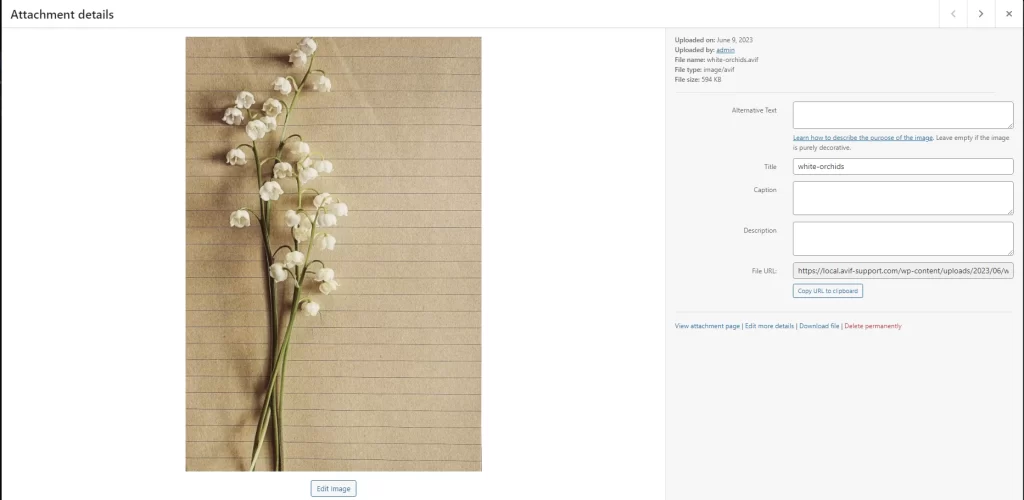
Once you’ve added this code, you will be able to upload AVIF images to your WordPress site. However, you will encounter additional issues after the upload process.
Empty Attachment Page
After uploading an AVIF image, you may notice that the view attachment page appears empty.
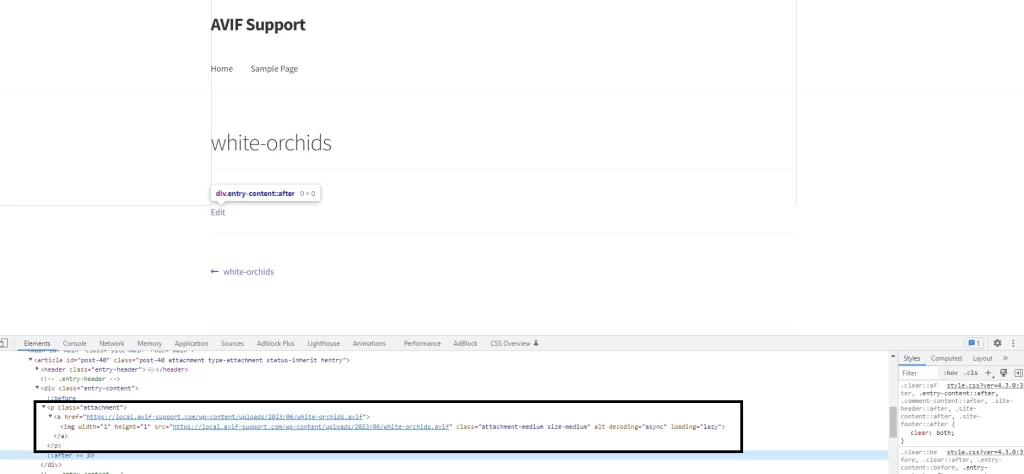
Well, the image is there, but the problem is the image width and height is 1px!!
If you check the image’s edit page, you will see that the dimensions are listed as 0x0. It’s broken.
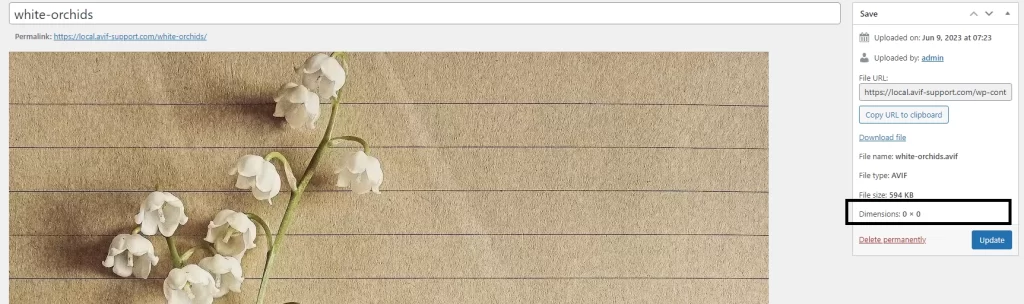
So, What went wrong here? the route cause of this issue is that WordPress relies on getimagesize() function to obtain the image dimensions before generating the image metadata, but the function returns incorrect width and height ( 0 pixels ) for AVIF images in PHP version prior to 8.2
To resolve this problem, we need to intercept the image’s metadata generation and use an alternative method to obtain the image correct dimensions.
Here’s a code snippet that accomplishes this:
add_filter( 'wp_generate_attachment_metadata', 'fix_avif_images', 1, 3 );
function fix_avif_images( $metadata, $attachment_id, $context ) {
if ( empty( $metadata ) ) {
return $metadata;
}
$attachemnt_post = get_post( $attachment_id );
if ( ! $attachemnt_post || is_wp_error( $attachemnt_post ) ) {
return $metadata;
}
if ( 'image/avif' !== $attachemnt_post->post_mime_type ) {
return $metadata;
}
if ( ( ! empty( $metadata['width'] ) && ( 0 !== $metadata['width'] ) ) && ( ! empty( $metadata['height'] ) && 0 !== $metadata['height'] ) ) {
return $metadata;
}
$file = get_attached_file( $attachment_id );
if ( ! $file ) {
return $metadata;
}
if ( empty( $metadata['width'] ) ) {
$metadata['width'] = 0;
}
if ( empty( $metadata['height'] ) ) {
$metadata['height'] = 0;
}
if ( empty( $metadata['file'] ) ) {
$metadata['file'] = _wp_relative_upload_path( $file );
}
if ( empty( $metadata['sizes'] ) ) {
$metadata['sizes'] = array();
}
try {
$imgick = new \Imagick( $file );
$img_dim = $imgick->getImageGeometry();
$imgick->clear();
$metadata['width'] = $img_dim['width'];
$metadata['height'] = $img_dim['height'];
} catch ( \Exception $e ) {
}
return $metadata;
}
in this code, we hook into the image metadata generation process before saving it and getting the image dimensions using Imagick
after uploading an AVIF image, The dimensions should be fixed and the image is displayable at the image view page.
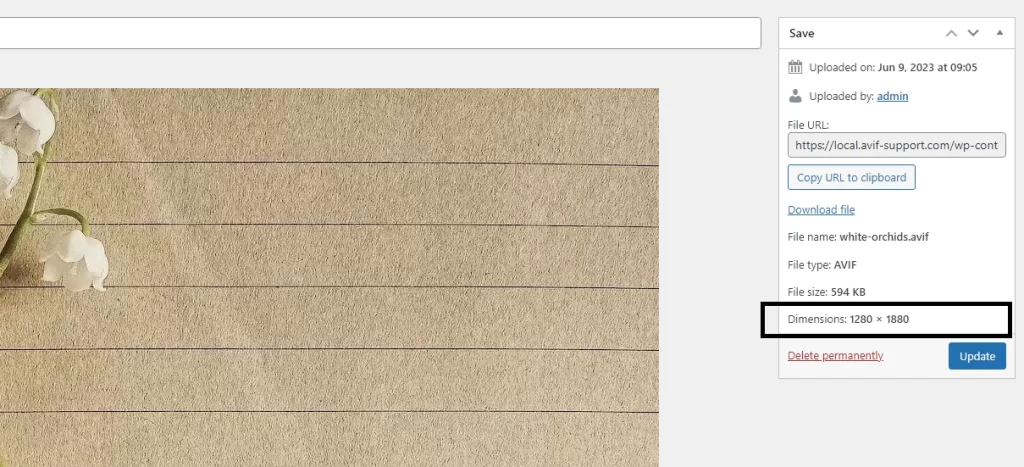
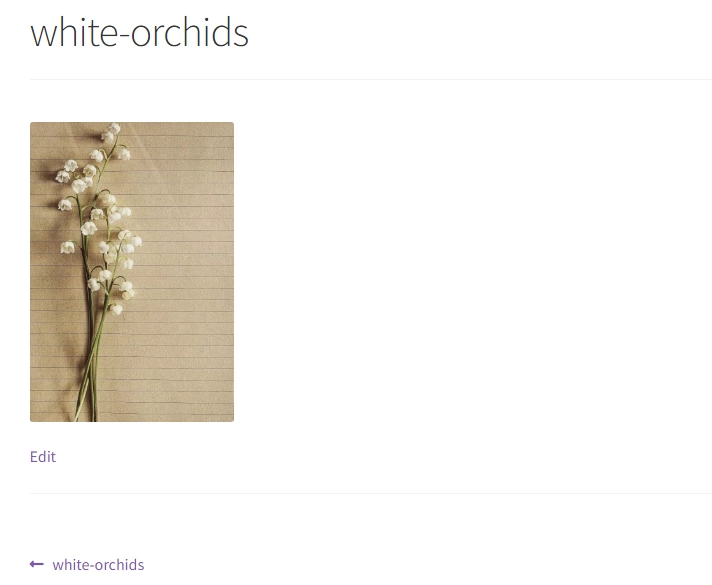
Sub-sizes
At this point,you will be able to to upload AVIF images and fix their dimensions. however, you will notice that WordPress doesn’t generate sub-sizes. This is because AVIF format still not registered at the sub-sizes generation process.
To address this issue, we need to register the AVIF format at the appropriate filter hooks:
add_filter( 'getimagesize_mimes_to_exts', 'filter_mime_to_exts', 1000, 1 );
add_filter( 'mime_types', 'filter_mime_types', 1000, 1 );
add_filter( 'file_is_displayable_image', 'fix_avif_displayable', 1000, 2 );
function filter_mime_to_exts( $mime_to_exsts ) {
$mime_to_exsts['image/avif'] = 'avif';
return $mime_to_exsts;
}
function filter_mime_types( $mimes ) {
$mimes['avif'] = 'image/avif';
return $mimes;
}
function fix_avif_displayable( $result, $path ) {
// Pypass avif.
if ( str_ends_with( $path, '.avif' ) ) {
return true;
}
return $result;
}
By adding these code snippets, we register the AVIF format for sub-size generation in WordPress. After uploading an AVIF image, the sub-sizes should be generated as expected.
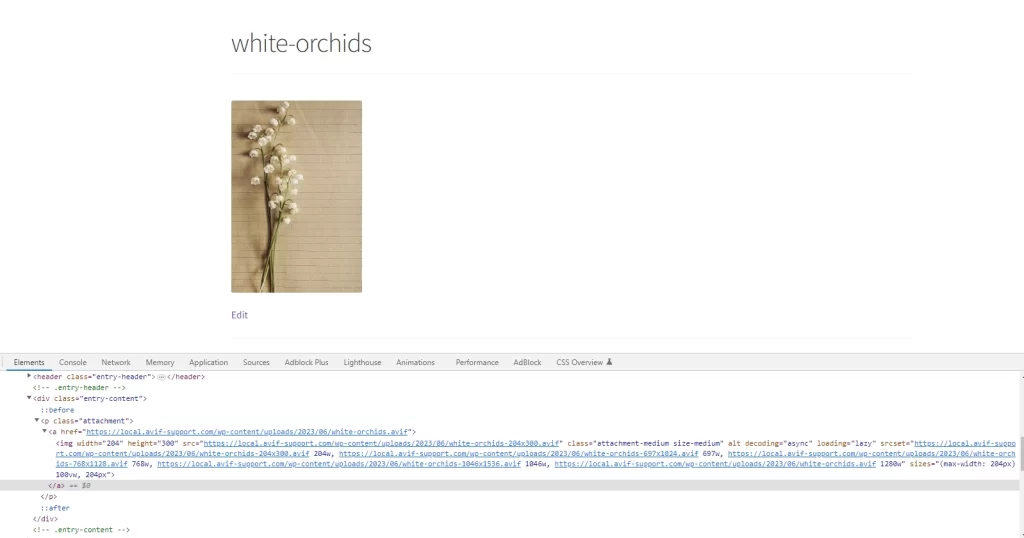
Reaching this point, you can upload AVIF images same as other image formats.
It’s worth noting that if you are hosting your website on a shared hosting platform with outdated GD and Imagick libraries that do not support the AVIF format
You will be able to upload the AVIF image though, but you can’t generate sub-sizes. you may encounter additional issues. Resolving such problems requires more comprehensive solutions beyond these code snippets. We’ve built the AVIF Support plugin where we combined all the aforementioned fixes beside other related low hosting issues.
Is AVIF worth it?
You may wonder if AVIF is truly worth adopting, considering that many people believe WEBP is sufficient for web use.
We conducted numerous tests, particularly converting from WEBP and other formats to AVIF using WP Image Converter plugin and AVIF consistently outperformed other formats.
In some cases, AVIF achieved astonishing results as demonstrated by the example below.
The image size was reduced by over %97 from 1.4 MB to 49.0 KB
WEBP
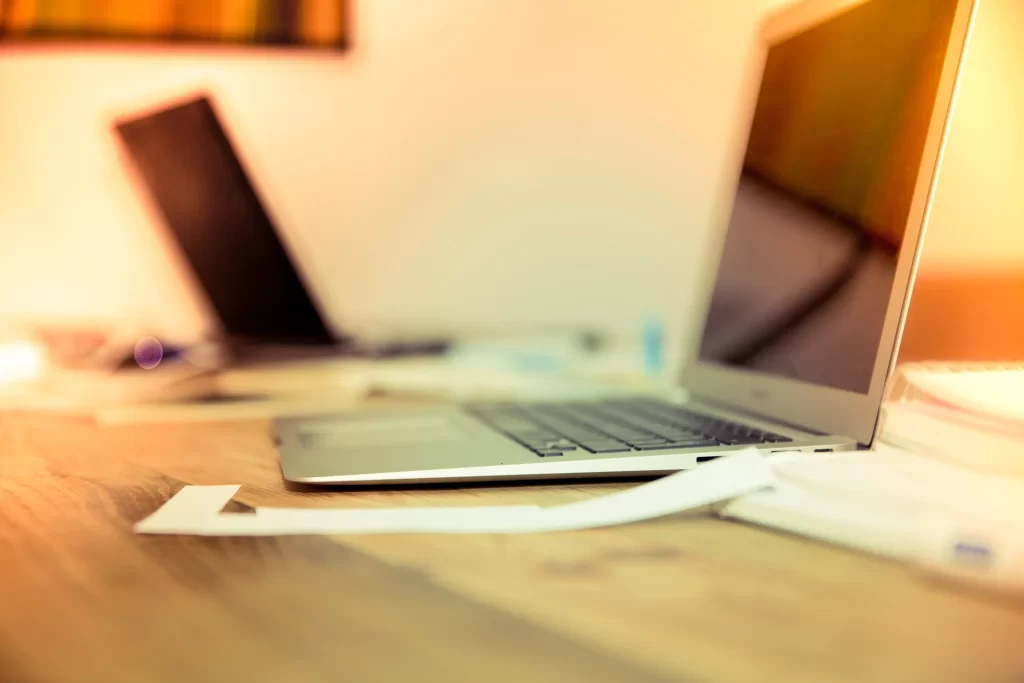
AVIF
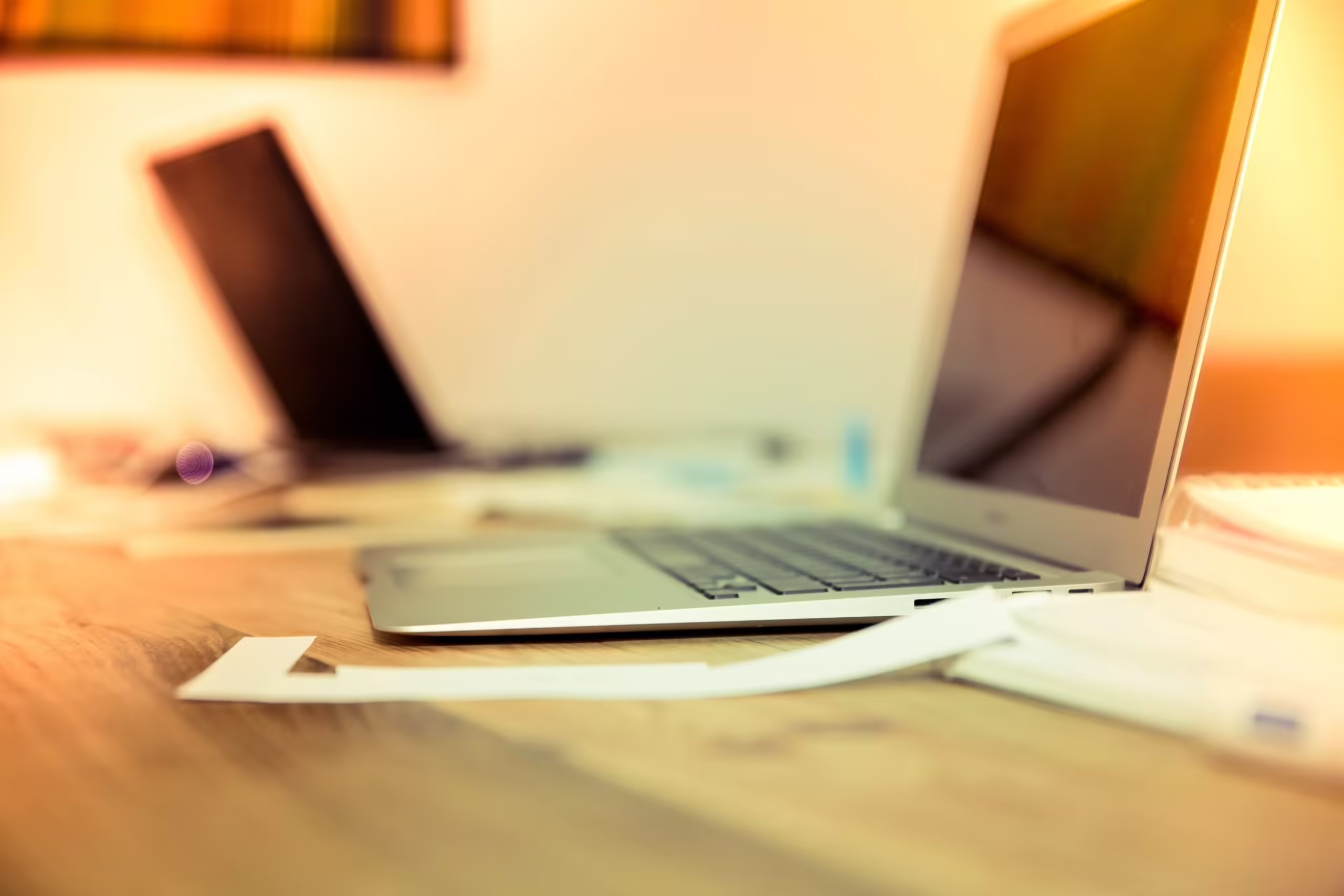
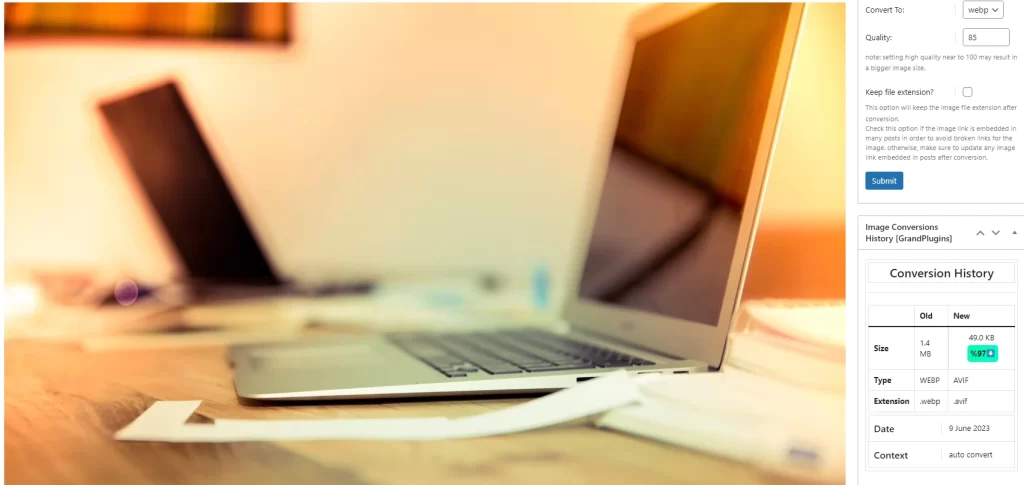
Sub-sizes
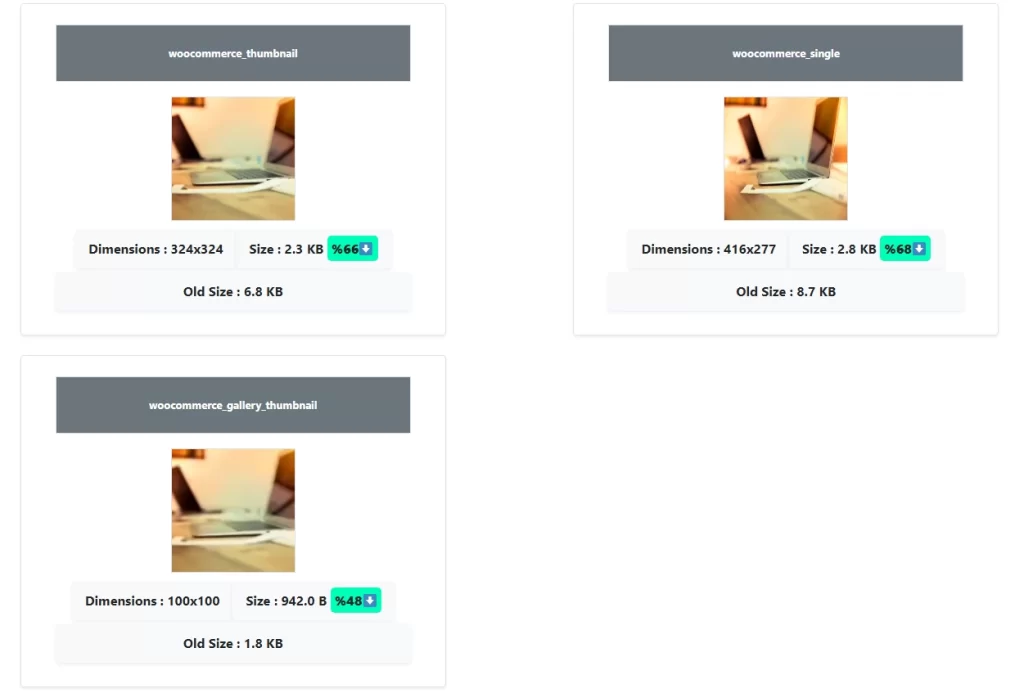
AVIF also outperformed WEBP when converting from different file types.
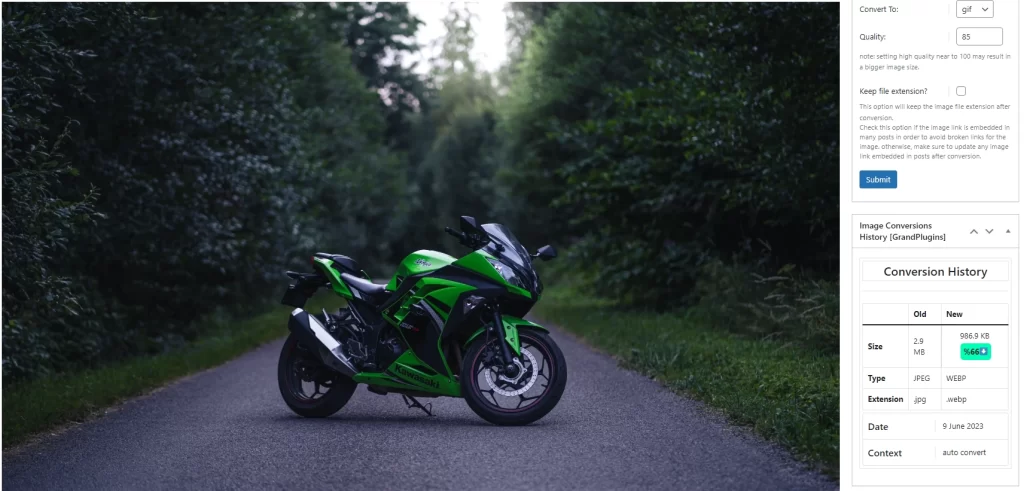
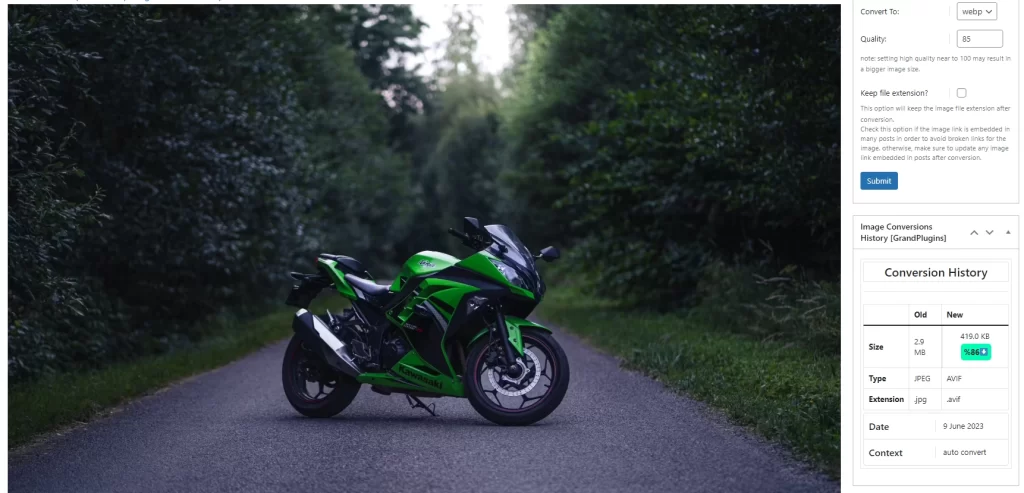
Considering the widespread support for AVIF in major browsers (Chrome, Firefox, Opera) and the increasing adoption of PHP versions 8.2 and above, AVIF is undoubtedly a format worth considering. It is likely to surpass other formats in the future, given its impressive compression capabilities and broad compatibility.
Thank you so much, very valuable knowledge!! 🙂
i am not sure i can not upload AVIF
I copy all code above in function.php does not allow me AVIF uplpad says
Screen is not problem no error.
Help me please.
Please, try with our plugin.
https://wordpress.org/plugins/avif-support/
let us know if you had any errors
Regards!
is there any way not plugin
i am scared to use plugin..
HI thanks for heloing me
so that code does not work? i delate.
but is there any way no plugin ?
Hi,
sometimes the snippet code won’t work. It depends on hosting setup, PHP version, and installed image processing library ( GD | Imagick ). Hard to tell where the issue is. It takes more than a snippet code to make some checks to avoid bitfalls in older PHP versions and WP lack of support to AVIF.
The plugin is simple. no worries 😀
This blog post hit all the right notes!