How To Remove WooCommerce Blocks Assets
If you upgraded WooCommerce plugin to 8.0 or beyond, you might have noticed a peculiar behavior. The plugin, in this update, enqueues all of its Gutenberg blocks assets on every page, regardless of whether these blocks are used on the page or not.
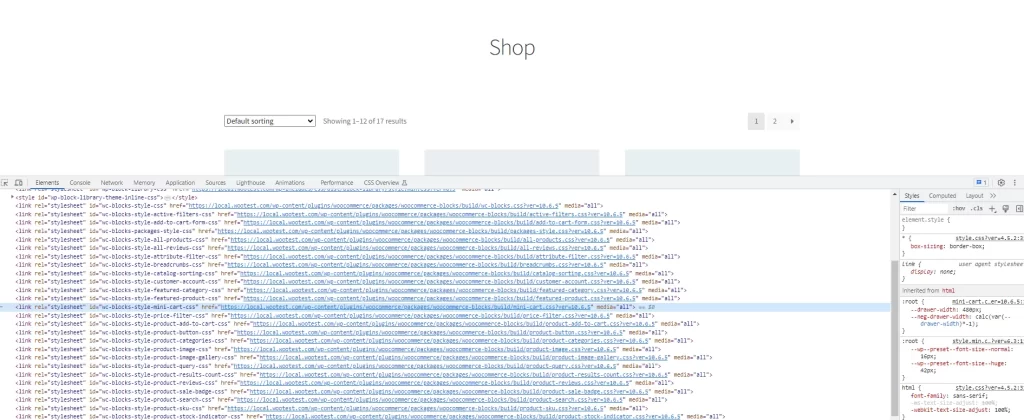
Upon closer inspection, you’ll discover about 30 or more CSS files included on any given page. Surprising, right? This could potentially slow down your site and create unnecessary clutter.
The Solution: Code Snippet
For those who don’t use WooCommerce Gutenberg Blocks on their websites, there’s a simple solution to remove these unnecessary asset files. Below, we provide a helpful code snippet that can remove all these assets:
add_action( 'wp_enqueue_scripts', 'gpls_snippet_remove_woo_blocks_assets', PHP_INT_MAX );
/**
* Remove enqueued WooCommerce Blocks Assets.
*
* @return void
*/
function gpls_snippet_remove_woo_blocks_assets() {
global $wp_styles;
foreach ( $wp_styles->queue as $index => $handle_name ) {
if ( str_starts_with( $handle_name, 'wc-blocks-' ) ) {
unset( $wp_styles->queue[ $index ] );
}
}
}
Please note, this snippet will prevent all WooCommerce blocks CSS files from loading. If you rely on using WooCommerce blocks on specific pages, you will need to modify the snippet with appropriate conditions to bypass the corresponding block’s CSS file.
Where to Place the Code Snippet
In order to implement this solution, you will need to place the code snippet in your theme’s functions.php
file. You can locate this file in your theme’s directory (/wp-content/themes/your-theme-name/
) on your server.
However, be aware that any changes you make to the theme’s files will be lost when you update the theme. To prevent this, it’s recommended to use a child theme or a custom plugin. This allows you to make changes without affecting the parent theme and prevents your modifications from being overwritten during updates.
Conclusion
The main aim here is to optimize your WooCommerce site by avoiding the unnecessary loading of assets. This not only speeds up your site but also improves the user experience, which is a key factor in SEO ranking.
Until this issue is fixed, by using the code snippet provided, you can efficiently manage your site’s resources, ensuring that only WooCommerce necessary assets are loaded on each page.